class cv::RotatedRect
Overview
The class represents rotated (i.e. not up-right) rectangles on a plane. Moreā¦
#include <types.hpp> class RotatedRect { public: // fields float angle; Point2f center; Size2f size; // construction RotatedRect(); RotatedRect( const Point2f& center, const Size2f& size, float angle ); RotatedRect( const Point2f& point1, const Point2f& point2, const Point2f& point3 ); // methods Rect boundingRect() const; Rect_<float> boundingRect2f() const; void points(Point2f pts []) const; };
Detailed Documentation
The class represents rotated (i.e. not up-right) rectangles on a plane.
Each rectangle is specified by the center point (mass center), length of each side (represented by cv::Size2f structure) and the rotation angle in degrees.
The sample below demonstrates how to use RotatedRect :
Mat image(200, 200, CV_8UC3, Scalar(0)); RotatedRect rRect = RotatedRect(Point2f(100,100), Size2f(100,50), 30); Point2f vertices[4]; rRect.points(vertices); for (int i = 0; i < 4; i++) line(image, vertices[i], vertices[(i+1)%4], Scalar(0,255,0)); Rect brect = rRect.boundingRect(); rectangle(image, brect, Scalar(255,0,0)); imshow("rectangles", image); waitKey(0);
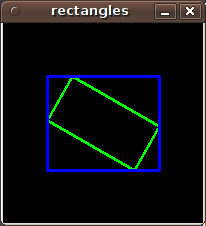
See also:
CamShift, fitEllipse, minAreaRect, CvBox2D
Construction
RotatedRect()
various constructors
RotatedRect( const Point2f& center, const Size2f& size, float angle )
Parameters:
center | The rectangle mass center. |
size | Width and height of the rectangle. |
angle | The rotation angle in a clockwise direction. When the angle is 0, 90, 180, 270 etc., the rectangle becomes an up-right rectangle. |
RotatedRect( const Point2f& point1, const Point2f& point2, const Point2f& point3 )
Any 3 end points of the RotatedRect. They must be given in order (either clockwise or anticlockwise).
Methods
Rect boundingRect() const
returns the minimal up-right integer rectangle containing the rotated rectangle
Rect_<float> boundingRect2f() const
returns the minimal (exact) floating point rectangle containing the rotated rectangle, not intended for use with images
void points(Point2f pts []) const
returns 4 vertices of the rectangle
Parameters:
pts | The points array for storing rectangle vertices. |