class cv::LineSegmentDetector
Overview
Line segment detector class. Moreā¦
#include <imgproc.hpp> class LineSegmentDetector: public cv::Algorithm { public: // methods virtual int compareSegments( const Size& size, InputArray lines1, InputArray lines2, InputOutputArray _image = noArray() ) = 0; virtual void detect( InputArray _image, OutputArray _lines, OutputArray width = noArray(), OutputArray prec = noArray(), OutputArray nfa = noArray() ) = 0; virtual void drawSegments( InputOutputArray _image, InputArray lines ) = 0; };
Inherited Members
public: // methods virtual void clear(); virtual bool empty() const; virtual String getDefaultName() const; virtual void read(const FileNode& fn); virtual void save(const String& filename) const; virtual void write(FileStorage& fs) const; template <typename _Tp> static Ptr<_Tp> load( const String& filename, const String& objname = String() ); template <typename _Tp> static Ptr<_Tp> loadFromString( const String& strModel, const String& objname = String() ); template <typename _Tp> static Ptr<_Tp> read(const FileNode& fn); protected: // methods void writeFormat(FileStorage& fs) const;
Detailed Documentation
Line segment detector class.
following the algorithm described at [89].
Methods
virtual int compareSegments( const Size& size, InputArray lines1, InputArray lines2, InputOutputArray _image = noArray() ) = 0
Draws two groups of lines in blue and red, counting the non overlapping (mismatching) pixels.
Parameters:
size | The size of the image, where lines1 and lines2 were found. |
lines1 | The first group of lines that needs to be drawn. It is visualized in blue color. |
lines2 | The second group of lines. They visualized in red color. |
_image | Optional image, where the lines will be drawn. The image should be color(3-channel) in order for lines1 and lines2 to be drawn in the above mentioned colors. |
virtual void detect( InputArray _image, OutputArray _lines, OutputArray width = noArray(), OutputArray prec = noArray(), OutputArray nfa = noArray() ) = 0
Finds lines in the input image.
This is the output of the default parameters of the algorithm on the above shown image.
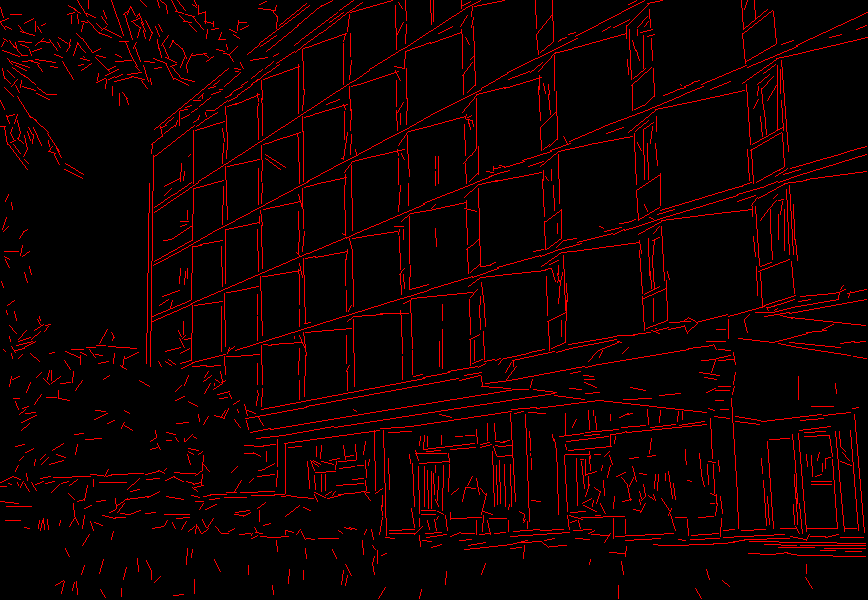
Parameters:
_image | A grayscale (CV_8UC1) input image. If only a roi needs to be selected, use: lsd_ptr-\>detect(image(roi), lines, ...); lines += Scalar(roi.x, roi.y, roi.x, roi.y); |
_lines | A vector of Vec4i or Vec4f elements specifying the beginning and ending point of a line. Where Vec4i/Vec4f is (x1, y1, x2, y2), point 1 is the start, point 2 - end. Returned lines are strictly oriented depending on the gradient. |
width | Vector of widths of the regions, where the lines are found. E.g. Width of line. |
prec | Vector of precisions with which the lines are found. |
nfa | Vector containing number of false alarms in the line region, with precision of 10%. The bigger the value, logarithmically better the detection.
|
virtual void drawSegments( InputOutputArray _image, InputArray lines ) = 0
Draws the line segments on a given image.
Parameters:
_image | The image, where the liens will be drawn. Should be bigger or equal to the image, where the lines were found. |
lines | A vector of the lines that needed to be drawn. |