C API
Overview
// typedefs typedef struct _CvContourScanner* CvContourScanner; typedef float (*CvDistanceFunction)( const float *a, const float *b, void *user_param ); // enums enum { @79::CV_GAUSSIAN_5x5 = 7, }; enum { @80::CV_SCHARR =-1, @80::CV_MAX_SOBEL_KSIZE =7, }; enum { @81::CV_BGR2BGRA =0, @81::CV_RGB2RGBA =CV_BGR2BGRA, @81::CV_BGRA2BGR =1, @81::CV_RGBA2RGB =CV_BGRA2BGR, @81::CV_BGR2RGBA =2, @81::CV_RGB2BGRA =CV_BGR2RGBA, @81::CV_RGBA2BGR =3, @81::CV_BGRA2RGB =CV_RGBA2BGR, @81::CV_BGR2RGB =4, @81::CV_RGB2BGR =CV_BGR2RGB, @81::CV_BGRA2RGBA =5, @81::CV_RGBA2BGRA =CV_BGRA2RGBA, @81::CV_BGR2GRAY =6, @81::CV_RGB2GRAY =7, @81::CV_GRAY2BGR =8, @81::CV_GRAY2RGB =CV_GRAY2BGR, @81::CV_GRAY2BGRA =9, @81::CV_GRAY2RGBA =CV_GRAY2BGRA, @81::CV_BGRA2GRAY =10, @81::CV_RGBA2GRAY =11, @81::CV_BGR2BGR565 =12, @81::CV_RGB2BGR565 =13, @81::CV_BGR5652BGR =14, @81::CV_BGR5652RGB =15, @81::CV_BGRA2BGR565 =16, @81::CV_RGBA2BGR565 =17, @81::CV_BGR5652BGRA =18, @81::CV_BGR5652RGBA =19, @81::CV_GRAY2BGR565 =20, @81::CV_BGR5652GRAY =21, @81::CV_BGR2BGR555 =22, @81::CV_RGB2BGR555 =23, @81::CV_BGR5552BGR =24, @81::CV_BGR5552RGB =25, @81::CV_BGRA2BGR555 =26, @81::CV_RGBA2BGR555 =27, @81::CV_BGR5552BGRA =28, @81::CV_BGR5552RGBA =29, @81::CV_GRAY2BGR555 =30, @81::CV_BGR5552GRAY =31, @81::CV_BGR2XYZ =32, @81::CV_RGB2XYZ =33, @81::CV_XYZ2BGR =34, @81::CV_XYZ2RGB =35, @81::CV_BGR2YCrCb =36, @81::CV_RGB2YCrCb =37, @81::CV_YCrCb2BGR =38, @81::CV_YCrCb2RGB =39, @81::CV_BGR2HSV =40, @81::CV_RGB2HSV =41, @81::CV_BGR2Lab =44, @81::CV_RGB2Lab =45, @81::CV_BayerBG2BGR =46, @81::CV_BayerGB2BGR =47, @81::CV_BayerRG2BGR =48, @81::CV_BayerGR2BGR =49, @81::CV_BayerBG2RGB =CV_BayerRG2BGR, @81::CV_BayerGB2RGB =CV_BayerGR2BGR, @81::CV_BayerRG2RGB =CV_BayerBG2BGR, @81::CV_BayerGR2RGB =CV_BayerGB2BGR, @81::CV_BGR2Luv =50, @81::CV_RGB2Luv =51, @81::CV_BGR2HLS =52, @81::CV_RGB2HLS =53, @81::CV_HSV2BGR =54, @81::CV_HSV2RGB =55, @81::CV_Lab2BGR =56, @81::CV_Lab2RGB =57, @81::CV_Luv2BGR =58, @81::CV_Luv2RGB =59, @81::CV_HLS2BGR =60, @81::CV_HLS2RGB =61, @81::CV_BayerBG2BGR_VNG =62, @81::CV_BayerGB2BGR_VNG =63, @81::CV_BayerRG2BGR_VNG =64, @81::CV_BayerGR2BGR_VNG =65, @81::CV_BayerBG2RGB_VNG =CV_BayerRG2BGR_VNG, @81::CV_BayerGB2RGB_VNG =CV_BayerGR2BGR_VNG, @81::CV_BayerRG2RGB_VNG =CV_BayerBG2BGR_VNG, @81::CV_BayerGR2RGB_VNG =CV_BayerGB2BGR_VNG, @81::CV_BGR2HSV_FULL = 66, @81::CV_RGB2HSV_FULL = 67, @81::CV_BGR2HLS_FULL = 68, @81::CV_RGB2HLS_FULL = 69, @81::CV_HSV2BGR_FULL = 70, @81::CV_HSV2RGB_FULL = 71, @81::CV_HLS2BGR_FULL = 72, @81::CV_HLS2RGB_FULL = 73, @81::CV_LBGR2Lab = 74, @81::CV_LRGB2Lab = 75, @81::CV_LBGR2Luv = 76, @81::CV_LRGB2Luv = 77, @81::CV_Lab2LBGR = 78, @81::CV_Lab2LRGB = 79, @81::CV_Luv2LBGR = 80, @81::CV_Luv2LRGB = 81, @81::CV_BGR2YUV = 82, @81::CV_RGB2YUV = 83, @81::CV_YUV2BGR = 84, @81::CV_YUV2RGB = 85, @81::CV_BayerBG2GRAY = 86, @81::CV_BayerGB2GRAY = 87, @81::CV_BayerRG2GRAY = 88, @81::CV_BayerGR2GRAY = 89, @81::CV_YUV2RGB_NV12 = 90, @81::CV_YUV2BGR_NV12 = 91, @81::CV_YUV2RGB_NV21 = 92, @81::CV_YUV2BGR_NV21 = 93, @81::CV_YUV420sp2RGB = CV_YUV2RGB_NV21, @81::CV_YUV420sp2BGR = CV_YUV2BGR_NV21, @81::CV_YUV2RGBA_NV12 = 94, @81::CV_YUV2BGRA_NV12 = 95, @81::CV_YUV2RGBA_NV21 = 96, @81::CV_YUV2BGRA_NV21 = 97, @81::CV_YUV420sp2RGBA = CV_YUV2RGBA_NV21, @81::CV_YUV420sp2BGRA = CV_YUV2BGRA_NV21, @81::CV_YUV2RGB_YV12 = 98, @81::CV_YUV2BGR_YV12 = 99, @81::CV_YUV2RGB_IYUV = 100, @81::CV_YUV2BGR_IYUV = 101, @81::CV_YUV2RGB_I420 = CV_YUV2RGB_IYUV, @81::CV_YUV2BGR_I420 = CV_YUV2BGR_IYUV, @81::CV_YUV420p2RGB = CV_YUV2RGB_YV12, @81::CV_YUV420p2BGR = CV_YUV2BGR_YV12, @81::CV_YUV2RGBA_YV12 = 102, @81::CV_YUV2BGRA_YV12 = 103, @81::CV_YUV2RGBA_IYUV = 104, @81::CV_YUV2BGRA_IYUV = 105, @81::CV_YUV2RGBA_I420 = CV_YUV2RGBA_IYUV, @81::CV_YUV2BGRA_I420 = CV_YUV2BGRA_IYUV, @81::CV_YUV420p2RGBA = CV_YUV2RGBA_YV12, @81::CV_YUV420p2BGRA = CV_YUV2BGRA_YV12, @81::CV_YUV2GRAY_420 = 106, @81::CV_YUV2GRAY_NV21 = CV_YUV2GRAY_420, @81::CV_YUV2GRAY_NV12 = CV_YUV2GRAY_420, @81::CV_YUV2GRAY_YV12 = CV_YUV2GRAY_420, @81::CV_YUV2GRAY_IYUV = CV_YUV2GRAY_420, @81::CV_YUV2GRAY_I420 = CV_YUV2GRAY_420, @81::CV_YUV420sp2GRAY = CV_YUV2GRAY_420, @81::CV_YUV420p2GRAY = CV_YUV2GRAY_420, @81::CV_YUV2RGB_UYVY = 107, @81::CV_YUV2BGR_UYVY = 108, @81::CV_YUV2RGB_Y422 = CV_YUV2RGB_UYVY, @81::CV_YUV2BGR_Y422 = CV_YUV2BGR_UYVY, @81::CV_YUV2RGB_UYNV = CV_YUV2RGB_UYVY, @81::CV_YUV2BGR_UYNV = CV_YUV2BGR_UYVY, @81::CV_YUV2RGBA_UYVY = 111, @81::CV_YUV2BGRA_UYVY = 112, @81::CV_YUV2RGBA_Y422 = CV_YUV2RGBA_UYVY, @81::CV_YUV2BGRA_Y422 = CV_YUV2BGRA_UYVY, @81::CV_YUV2RGBA_UYNV = CV_YUV2RGBA_UYVY, @81::CV_YUV2BGRA_UYNV = CV_YUV2BGRA_UYVY, @81::CV_YUV2RGB_YUY2 = 115, @81::CV_YUV2BGR_YUY2 = 116, @81::CV_YUV2RGB_YVYU = 117, @81::CV_YUV2BGR_YVYU = 118, @81::CV_YUV2RGB_YUYV = CV_YUV2RGB_YUY2, @81::CV_YUV2BGR_YUYV = CV_YUV2BGR_YUY2, @81::CV_YUV2RGB_YUNV = CV_YUV2RGB_YUY2, @81::CV_YUV2BGR_YUNV = CV_YUV2BGR_YUY2, @81::CV_YUV2RGBA_YUY2 = 119, @81::CV_YUV2BGRA_YUY2 = 120, @81::CV_YUV2RGBA_YVYU = 121, @81::CV_YUV2BGRA_YVYU = 122, @81::CV_YUV2RGBA_YUYV = CV_YUV2RGBA_YUY2, @81::CV_YUV2BGRA_YUYV = CV_YUV2BGRA_YUY2, @81::CV_YUV2RGBA_YUNV = CV_YUV2RGBA_YUY2, @81::CV_YUV2BGRA_YUNV = CV_YUV2BGRA_YUY2, @81::CV_YUV2GRAY_UYVY = 123, @81::CV_YUV2GRAY_YUY2 = 124, @81::CV_YUV2GRAY_Y422 = CV_YUV2GRAY_UYVY, @81::CV_YUV2GRAY_UYNV = CV_YUV2GRAY_UYVY, @81::CV_YUV2GRAY_YVYU = CV_YUV2GRAY_YUY2, @81::CV_YUV2GRAY_YUYV = CV_YUV2GRAY_YUY2, @81::CV_YUV2GRAY_YUNV = CV_YUV2GRAY_YUY2, @81::CV_RGBA2mRGBA = 125, @81::CV_mRGBA2RGBA = 126, @81::CV_RGB2YUV_I420 = 127, @81::CV_BGR2YUV_I420 = 128, @81::CV_RGB2YUV_IYUV = CV_RGB2YUV_I420, @81::CV_BGR2YUV_IYUV = CV_BGR2YUV_I420, @81::CV_RGBA2YUV_I420 = 129, @81::CV_BGRA2YUV_I420 = 130, @81::CV_RGBA2YUV_IYUV = CV_RGBA2YUV_I420, @81::CV_BGRA2YUV_IYUV = CV_BGRA2YUV_I420, @81::CV_RGB2YUV_YV12 = 131, @81::CV_BGR2YUV_YV12 = 132, @81::CV_RGBA2YUV_YV12 = 133, @81::CV_BGRA2YUV_YV12 = 134, @81::CV_BayerBG2BGR_EA = 135, @81::CV_BayerGB2BGR_EA = 136, @81::CV_BayerRG2BGR_EA = 137, @81::CV_BayerGR2BGR_EA = 138, @81::CV_BayerBG2RGB_EA = CV_BayerRG2BGR_EA, @81::CV_BayerGB2RGB_EA = CV_BayerGR2BGR_EA, @81::CV_BayerRG2RGB_EA = CV_BayerBG2BGR_EA, @81::CV_BayerGR2RGB_EA = CV_BayerGB2BGR_EA, @81::CV_BayerBG2BGRA =139, @81::CV_BayerGB2BGRA =140, @81::CV_BayerRG2BGRA =141, @81::CV_BayerGR2BGRA =142, @81::CV_BayerBG2RGBA =CV_BayerRG2BGRA, @81::CV_BayerGB2RGBA =CV_BayerGR2BGRA, @81::CV_BayerRG2RGBA =CV_BayerBG2BGRA, @81::CV_BayerGR2RGBA =CV_BayerGB2BGRA, @81::CV_COLORCVT_MAX = 143, }; enum { @82::CV_INTER_NN =0, @82::CV_INTER_LINEAR =1, @82::CV_INTER_CUBIC =2, @82::CV_INTER_AREA =3, @82::CV_INTER_LANCZOS4 =4, }; enum { @83::CV_WARP_FILL_OUTLIERS =8, @83::CV_WARP_INVERSE_MAP =16, }; enum { @84::CV_MOP_ERODE =0, @84::CV_MOP_DILATE =1, @84::CV_MOP_OPEN =2, @84::CV_MOP_CLOSE =3, @84::CV_MOP_GRADIENT =4, @84::CV_MOP_TOPHAT =5, @84::CV_MOP_BLACKHAT =6, }; enum { @85::CV_TM_SQDIFF =0, @85::CV_TM_SQDIFF_NORMED =1, @85::CV_TM_CCORR =2, @85::CV_TM_CCORR_NORMED =3, @85::CV_TM_CCOEFF =4, @85::CV_TM_CCOEFF_NORMED =5, }; enum { @86::CV_RETR_EXTERNAL =0, @86::CV_RETR_LIST =1, @86::CV_RETR_CCOMP =2, @86::CV_RETR_TREE =3, @86::CV_RETR_FLOODFILL =4, }; enum { @87::CV_CHAIN_CODE =0, @87::CV_CHAIN_APPROX_NONE =1, @87::CV_CHAIN_APPROX_SIMPLE =2, @87::CV_CHAIN_APPROX_TC89_L1 =3, @87::CV_CHAIN_APPROX_TC89_KCOS =4, @87::CV_LINK_RUNS =5, }; enum { @88::CV_POLY_APPROX_DP = 0, }; enum { @89::CV_CONTOURS_MATCH_I1 =1, @89::CV_CONTOURS_MATCH_I2 =2, @89::CV_CONTOURS_MATCH_I3 =3, }; enum { @90::CV_CLOCKWISE =1, @90::CV_COUNTER_CLOCKWISE =2, }; enum { @91::CV_COMP_CORREL =0, @91::CV_COMP_CHISQR =1, @91::CV_COMP_INTERSECT =2, @91::CV_COMP_BHATTACHARYYA =3, @91::CV_COMP_HELLINGER =CV_COMP_BHATTACHARYYA, @91::CV_COMP_CHISQR_ALT =4, @91::CV_COMP_KL_DIV =5, }; enum { @92::CV_DIST_MASK_3 =3, @92::CV_DIST_MASK_5 =5, @92::CV_DIST_MASK_PRECISE =0, }; enum { @93::CV_DIST_LABEL_CCOMP = 0, @93::CV_DIST_LABEL_PIXEL = 1, }; enum { @94::CV_DIST_USER =-1, @94::CV_DIST_L1 =1, @94::CV_DIST_L2 =2, @94::CV_DIST_C =3, @94::CV_DIST_L12 =4, @94::CV_DIST_FAIR =5, @94::CV_DIST_WELSCH =6, @94::CV_DIST_HUBER =7, }; enum { @95::CV_THRESH_BINARY =0, @95::CV_THRESH_BINARY_INV =1, @95::CV_THRESH_TRUNC =2, @95::CV_THRESH_TOZERO =3, @95::CV_THRESH_TOZERO_INV =4, @95::CV_THRESH_MASK =7, @95::CV_THRESH_OTSU =8, @95::CV_THRESH_TRIANGLE =16, }; enum { @96::CV_ADAPTIVE_THRESH_MEAN_C =0, @96::CV_ADAPTIVE_THRESH_GAUSSIAN_C =1, }; enum { @97::CV_FLOODFILL_FIXED_RANGE =(1 <<16), @97::CV_FLOODFILL_MASK_ONLY =(1 <<17), }; enum { @98::CV_CANNY_L2_GRADIENT =(1 <<31), }; enum { @99::CV_HOUGH_STANDARD =0, @99::CV_HOUGH_PROBABILISTIC =1, @99::CV_HOUGH_MULTI_SCALE =2, @99::CV_HOUGH_GRADIENT =3, }; enum MorphShapes_c; enum SmoothMethod_c; // structs struct CvChainPtReader; struct CvConnectedComp; struct CvConvexityDefect; struct CvFont; struct CvHuMoments; struct CvMoments; // global functions CvMat* cv2DRotationMatrix( CvPoint2D32f center, double angle, double scale, CvMat* map_matrix ); void cvAcc( const CvArr* image, CvArr* sum, const CvArr* mask = NULL ); void cvAdaptiveThreshold( const CvArr* src, CvArr* dst, double max_value, int adaptive_method = CV_ADAPTIVE_THRESH_MEAN_C, int threshold_type = CV_THRESH_BINARY, int block_size = 3, double param1 = 5 ); CvSeq* cvApproxChains( CvSeq* src_seq, CvMemStorage* storage, int method = CV_CHAIN_APPROX_SIMPLE, double parameter = 0, int minimal_perimeter = 0, int recursive = 0 ); CvSeq* cvApproxPoly( const void* src_seq, int header_size, CvMemStorage* storage, int method, double eps, int recursive = 0 ); double cvArcLength( const void* curve, CvSlice slice = CV_WHOLE_SEQ, int is_closed = -1 ); CvRect cvBoundingRect( CvArr* points, int update = 0 ); void cvBoxPoints( CvBox2D box, CvPoint2D32f pt [4] ); void cvCalcArrBackProject( CvArr** image, CvArr* dst, const CvHistogram* hist ); void cvCalcArrBackProjectPatch( CvArr** image, CvArr* dst, CvSize range, CvHistogram* hist, int method, double factor ); void cvCalcArrHist( CvArr** arr, CvHistogram* hist, int accumulate = 0, const CvArr* mask = NULL ); void cvCalcBayesianProb( CvHistogram** src, int number, CvHistogram** dst ); float cvCalcEMD2( const CvArr* signature1, const CvArr* signature2, int distance_type, CvDistanceFunction distance_func = NULL, const CvArr* cost_matrix = NULL, CvArr* flow = NULL, float* lower_bound = NULL, void* userdata = NULL ); void cvCalcHist( IplImage** image, CvHistogram* hist, int accumulate = 0, const CvArr* mask = NULL ); void cvCalcProbDensity( const CvHistogram* hist1, const CvHistogram* hist2, CvHistogram* dst_hist, double scale = 255 ); void cvCanny( const CvArr* image, CvArr* edges, double threshold1, double threshold2, int aperture_size = 3 ); int cvCheckContourConvexity(const CvArr* contour); void cvCircle( CvArr* img, CvPoint center, int radius, CvScalar color, int thickness = 1, int line_type = 8, int shift = 0 ); void cvClearHist(CvHistogram* hist); int cvClipLine( CvSize img_size, CvPoint* pt1, CvPoint* pt2 ); CvScalar cvColorToScalar( double packed_color, int arrtype ); double cvCompareHist( const CvHistogram* hist1, const CvHistogram* hist2, int method ); double cvContourArea( const CvArr* contour, CvSlice slice = CV_WHOLE_SEQ, int oriented = 0 ); double cvContourPerimeter(const void* contour); void cvConvertMaps( const CvArr* mapx, const CvArr* mapy, CvArr* mapxy, CvArr* mapalpha ); CvSeq* cvConvexHull2( const CvArr* input, void* hull_storage = NULL, int orientation = CV_CLOCKWISE, int return_points = 0 ); CvSeq* cvConvexityDefects( const CvArr* contour, const CvArr* convexhull, CvMemStorage* storage = NULL ); void cvCopyHist( const CvHistogram* src, CvHistogram** dst ); void cvCopyMakeBorder( const CvArr* src, CvArr* dst, CvPoint offset, int bordertype, CvScalar value = cvScalarAll(0) ); void cvCornerEigenValsAndVecs( const CvArr* image, CvArr* eigenvv, int block_size, int aperture_size = 3 ); void cvCornerHarris( const CvArr* image, CvArr* harris_response, int block_size, int aperture_size = 3, double k = 0.04 ); void cvCornerMinEigenVal( const CvArr* image, CvArr* eigenval, int block_size, int aperture_size = 3 ); CvHistogram* cvCreateHist( int dims, int* sizes, int type, float** ranges = NULL, int uniform = 1 ); CvMat** cvCreatePyramid( const CvArr* img, int extra_layers, double rate, const CvSize* layer_sizes = 0, CvArr* bufarr = 0, int calc = 1, int filter = CV_GAUSSIAN_5x5 ); IplConvKernel* cvCreateStructuringElementEx( int cols, int rows, int anchor_x, int anchor_y, int shape, int* values = NULL ); void cvCvtColor( const CvArr* src, CvArr* dst, int code ); void cvDilate( const CvArr* src, CvArr* dst, IplConvKernel* element = NULL, int iterations = 1 ); void cvDistTransform( const CvArr* src, CvArr* dst, int distance_type = CV_DIST_L2, int mask_size = 3, const float* mask = NULL, CvArr* labels = NULL, int labelType = CV_DIST_LABEL_CCOMP ); void cvDrawContours( CvArr* img, CvSeq* contour, CvScalar external_color, CvScalar hole_color, int max_level, int thickness = 1, int line_type = 8, CvPoint offset = cvPoint(0, 0) ); void cvEllipse( CvArr* img, CvPoint center, CvSize axes, double angle, double start_angle, double end_angle, CvScalar color, int thickness = 1, int line_type = 8, int shift = 0 ); int cvEllipse2Poly( CvPoint center, CvSize axes, int angle, int arc_start, int arc_end, CvPoint* pts, int delta ); void cvEllipseBox( CvArr* img, CvBox2D box, CvScalar color, int thickness = 1, int line_type = 8, int shift = 0 ); CvSeq* cvEndFindContours(CvContourScanner* scanner); void cvEqualizeHist( const CvArr* src, CvArr* dst ); void cvErode( const CvArr* src, CvArr* dst, IplConvKernel* element = NULL, int iterations = 1 ); void cvFillConvexPoly( CvArr* img, const CvPoint* pts, int npts, CvScalar color, int line_type = 8, int shift = 0 ); void cvFillPoly( CvArr* img, CvPoint** pts, const int* npts, int contours, CvScalar color, int line_type = 8, int shift = 0 ); void cvFilter2D( const CvArr* src, CvArr* dst, const CvMat* kernel, CvPoint anchor = cvPoint(-1,-1) ); int cvFindContours( CvArr* image, CvMemStorage* storage, CvSeq** first_contour, int header_size = sizeof(CvContour), int mode = CV_RETR_LIST, int method = CV_CHAIN_APPROX_SIMPLE, CvPoint offset = cvPoint(0, 0) ); void cvFindCornerSubPix( const CvArr* image, CvPoint2D32f* corners, int count, CvSize win, CvSize zero_zone, CvTermCriteria criteria ); CvSeq* cvFindNextContour(CvContourScanner scanner); CvBox2D cvFitEllipse2(const CvArr* points); void cvFitLine( const CvArr* points, int dist_type, double param, double reps, double aeps, float* line ); void cvFloodFill( CvArr* image, CvPoint seed_point, CvScalar new_val, CvScalar lo_diff = cvScalarAll(0), CvScalar up_diff = cvScalarAll(0), CvConnectedComp* comp = NULL, int flags = 4, CvArr* mask = NULL ); CvFont cvFont( double scale, int thickness = 1 ); CvMat* cvGetAffineTransform( const CvPoint2D32f* src, const CvPoint2D32f* dst, CvMat* map_matrix ); double cvGetCentralMoment( CvMoments* moments, int x_order, int y_order ); void cvGetHuMoments( CvMoments* moments, CvHuMoments* hu_moments ); void cvGetMinMaxHistValue( const CvHistogram* hist, float* min_value, float* max_value, int* min_idx = NULL, int* max_idx = NULL ); double cvGetNormalizedCentralMoment( CvMoments* moments, int x_order, int y_order ); CvMat* cvGetPerspectiveTransform( const CvPoint2D32f* src, const CvPoint2D32f* dst, CvMat* map_matrix ); void cvGetQuadrangleSubPix( const CvArr* src, CvArr* dst, const CvMat* map_matrix ); void cvGetRectSubPix( const CvArr* src, CvArr* dst, CvPoint2D32f center ); double cvGetSpatialMoment( CvMoments* moments, int x_order, int y_order ); void cvGetTextSize( const char* text_string, const CvFont* font, CvSize* text_size, int* baseline ); void cvGoodFeaturesToTrack( const CvArr* image, CvArr* eig_image, CvArr* temp_image, CvPoint2D32f* corners, int* corner_count, double quality_level, double min_distance, const CvArr* mask = NULL, int block_size = 3, int use_harris = 0, double k = 0.04 ); CvSeq* cvHoughCircles( CvArr* image, void* circle_storage, int method, double dp, double min_dist, double param1 = 100, double param2 = 100, int min_radius = 0, int max_radius = 0 ); CvSeq* cvHoughLines2( CvArr* image, void* line_storage, int method, double rho, double theta, int threshold, double param1 = 0, double param2 = 0, double min_theta = 0, double max_theta = CV_PI ); void cvInitFont( CvFont* font, int font_face, double hscale, double vscale, double shear = 0, int thickness = 1, int line_type = 8 ); int cvInitLineIterator( const CvArr* image, CvPoint pt1, CvPoint pt2, CvLineIterator* line_iterator, int connectivity = 8, int left_to_right = 0 ); void cvInitUndistortMap( const CvMat* camera_matrix, const CvMat* distortion_coeffs, CvArr* mapx, CvArr* mapy ); void cvInitUndistortRectifyMap( const CvMat* camera_matrix, const CvMat* dist_coeffs, const CvMat* R, const CvMat* new_camera_matrix, CvArr* mapx, CvArr* mapy ); void cvIntegral( const CvArr* image, CvArr* sum, CvArr* sqsum = NULL, CvArr* tilted_sum = NULL ); void cvLaplace( const CvArr* src, CvArr* dst, int aperture_size = 3 ); void cvLine( CvArr* img, CvPoint pt1, CvPoint pt2, CvScalar color, int thickness = 1, int line_type = 8, int shift = 0 ); void cvLinearPolar( const CvArr* src, CvArr* dst, CvPoint2D32f center, double maxRadius, int flags = CV_INTER_LINEAR+CV_WARP_FILL_OUTLIERS ); void cvLogPolar( const CvArr* src, CvArr* dst, CvPoint2D32f center, double M, int flags = CV_INTER_LINEAR+CV_WARP_FILL_OUTLIERS ); CvHistogram* cvMakeHistHeaderForArray( int dims, int* sizes, CvHistogram* hist, float* data, float** ranges = NULL, int uniform = 1 ); double cvMatchShapes( const void* object1, const void* object2, int method, double parameter = 0 ); void cvMatchTemplate( const CvArr* image, const CvArr* templ, CvArr* result, int method ); CvRect cvMaxRect( const CvRect* rect1, const CvRect* rect2 ); CvBox2D cvMinAreaRect2( const CvArr* points, CvMemStorage* storage = NULL ); int cvMinEnclosingCircle( const CvArr* points, CvPoint2D32f* center, float* radius ); void cvMoments( const CvArr* arr, CvMoments* moments, int binary = 0 ); void cvMorphologyEx( const CvArr* src, CvArr* dst, CvArr* temp, IplConvKernel* element, int operation, int iterations = 1 ); void cvMultiplyAcc( const CvArr* image1, const CvArr* image2, CvArr* acc, const CvArr* mask = NULL ); void cvNormalizeHist( CvHistogram* hist, double factor ); double cvPointPolygonTest( const CvArr* contour, CvPoint2D32f pt, int measure_dist ); CvSeq* cvPointSeqFromMat( int seq_kind, const CvArr* mat, CvContour* contour_header, CvSeqBlock* block ); void cvPolyLine( CvArr* img, CvPoint** pts, const int* npts, int contours, int is_closed, CvScalar color, int thickness = 1, int line_type = 8, int shift = 0 ); void cvPreCornerDetect( const CvArr* image, CvArr* corners, int aperture_size = 3 ); void cvPutText( CvArr* img, const char* text, CvPoint org, const CvFont* font, CvScalar color ); void cvPyrDown( const CvArr* src, CvArr* dst, int filter = CV_GAUSSIAN_5x5 ); void cvPyrMeanShiftFiltering( const CvArr* src, CvArr* dst, double sp, double sr, int max_level = 1, CvTermCriteria termcrit = cvTermCriteria(CV_TERMCRIT_ITER+CV_TERMCRIT_EPS, 5, 1) ); void cvPyrUp( const CvArr* src, CvArr* dst, int filter = CV_GAUSSIAN_5x5 ); CvPoint cvReadChainPoint(CvChainPtReader* reader); void cvRectangle( CvArr* img, CvPoint pt1, CvPoint pt2, CvScalar color, int thickness = 1, int line_type = 8, int shift = 0 ); void cvRectangleR( CvArr* img, CvRect r, CvScalar color, int thickness = 1, int line_type = 8, int shift = 0 ); void cvReleaseHist(CvHistogram** hist); void cvReleasePyramid( CvMat*** pyramid, int extra_layers ); void cvReleaseStructuringElement(IplConvKernel** element); void cvRemap( const CvArr* src, CvArr* dst, const CvArr* mapx, const CvArr* mapy, int flags = CV_INTER_LINEAR+CV_WARP_FILL_OUTLIERS, CvScalar fillval = cvScalarAll(0) ); void cvResize( const CvArr* src, CvArr* dst, int interpolation = CV_INTER_LINEAR ); void cvRunningAvg( const CvArr* image, CvArr* acc, double alpha, const CvArr* mask = NULL ); int cvSampleLine( const CvArr* image, CvPoint pt1, CvPoint pt2, void* buffer, int connectivity = 8 ); void cvSetHistBinRanges( CvHistogram* hist, float** ranges, int uniform = 1 ); void cvSmooth( const CvArr* src, CvArr* dst, int smoothtype = CV_GAUSSIAN, int size1 = 3, int size2 = 0, double sigma1 = 0, double sigma2 = 0 ); void cvSobel( const CvArr* src, CvArr* dst, int xorder, int yorder, int aperture_size = 3 ); void cvSquareAcc( const CvArr* image, CvArr* sqsum, const CvArr* mask = NULL ); CvContourScanner cvStartFindContours( CvArr* image, CvMemStorage* storage, int header_size = sizeof(CvContour), int mode = CV_RETR_LIST, int method = CV_CHAIN_APPROX_SIMPLE, CvPoint offset = cvPoint(0, 0) ); void cvStartReadChainPoints( CvChain* chain, CvChainPtReader* reader ); void cvSubstituteContour( CvContourScanner scanner, CvSeq* new_contour ); void cvThreshHist( CvHistogram* hist, double threshold ); double cvThreshold( const CvArr* src, CvArr* dst, double threshold, double max_value, int threshold_type ); void cvUndistort2( const CvArr* src, CvArr* dst, const CvMat* camera_matrix, const CvMat* distortion_coeffs, const CvMat* new_camera_matrix = 0 ); void cvUndistortPoints( const CvMat* src, CvMat* dst, const CvMat* camera_matrix, const CvMat* dist_coeffs, const CvMat* R = 0, const CvMat* P = 0 ); void cvWarpAffine( const CvArr* src, CvArr* dst, const CvMat* map_matrix, int flags = CV_INTER_LINEAR+CV_WARP_FILL_OUTLIERS, CvScalar fillval = cvScalarAll(0) ); void cvWarpPerspective( const CvArr* src, CvArr* dst, const CvMat* map_matrix, int flags = CV_INTER_LINEAR+CV_WARP_FILL_OUTLIERS, CvScalar fillval = cvScalarAll(0) ); void cvWatershed( const CvArr* image, CvArr* markers ); // macros #define CV_AA #define CV_FILLED #define CV_FONT_HERSHEY_COMPLEX #define CV_FONT_HERSHEY_COMPLEX_SMALL #define CV_FONT_HERSHEY_DUPLEX #define CV_FONT_HERSHEY_PLAIN #define CV_FONT_HERSHEY_SCRIPT_COMPLEX #define CV_FONT_HERSHEY_SCRIPT_SIMPLEX #define CV_FONT_HERSHEY_SIMPLEX #define CV_FONT_HERSHEY_TRIPLEX #define CV_FONT_ITALIC #define CV_FONT_VECTOR0 #define CV_INIT_3X3_DELTAS( \ deltas, \ step, \ nch \ ) #define CV_NEXT_LINE_POINT(line_iterator) #define CV_RGB( \ r, \ g, \ b \ ) #define cvCalcBackProject( \ image, \ dst, \ hist \ ) #define cvCalcBackProjectPatch( \ image, \ dst, \ range, \ hist, \ method, \ factor \ ) #define cvDrawCircle #define cvDrawEllipse #define cvDrawLine #define cvDrawPolyLine #define cvDrawRect
Detailed Documentation
Enum Values
CV_CONTOURS_MATCH_I1
CV_CONTOURS_MATCH_I2
CV_CONTOURS_MATCH_I3
CV_DIST_USER
User defined distance
CV_DIST_L1
distance = |x1-x2| + |y1-y2|
CV_DIST_L2
the simple euclidean distance
CV_DIST_C
distance = max(|x1-x2|,|y1-y2|)
CV_DIST_L12
L1-L2 metric: distance = 2(sqrt(1+x*x/2) - 1))
CV_DIST_FAIR
distance = c^2(|x|/c-log(1+|x|/c)), c = 1.3998
CV_DIST_WELSCH
distance = c^2/2(1-exp(-(x/c)^2)), c = 2.9846
CV_DIST_HUBER
distance = |x|<c ? x^2/2 : c(|x|-c/2), c=1.345
CV_THRESH_BINARY
value = value > threshold ? max_value : 0
CV_THRESH_BINARY_INV
value = value > threshold ? 0 : max_value
CV_THRESH_TRUNC
value = value > threshold ? threshold : value
CV_THRESH_TOZERO
value = value > threshold ? value : 0
CV_THRESH_TOZERO_INV
value = value > threshold ? 0 : value
CV_THRESH_OTSU
use Otsu algorithm to choose the optimal threshold value; combine the flag with one of the above CV_THRESH_* values
CV_THRESH_TRIANGLE
use Triangle algorithm to choose the optimal threshold value; combine the flag with one of the above CV_THRESH_* values, but not with CV_THRESH_OTSU
Global Functions
CvMat* cv2DRotationMatrix( CvPoint2D32f center, double angle, double scale, CvMat* map_matrix )
Computes rotation_matrix matrix.
See also:
void cvAcc( const CvArr* image, CvArr* sum, const CvArr* mask = NULL )
Adds image to accumulator.
See also:
void cvAdaptiveThreshold( const CvArr* src, CvArr* dst, double max_value, int adaptive_method = CV_ADAPTIVE_THRESH_MEAN_C, int threshold_type = CV_THRESH_BINARY, int block_size = 3, double param1 = 5 )
Applies adaptive threshold to grayscale image.
The two parameters for methods CV_ADAPTIVE_THRESH_MEAN_C and CV_ADAPTIVE_THRESH_GAUSSIAN_C are: neighborhood size (3, 5, 7 etc.), and a constant subtracted from mean (…,-3,-2,-1,0,1,2,3,…)
See also:
CvSeq* cvApproxChains( CvSeq* src_seq, CvMemStorage* storage, int method = CV_CHAIN_APPROX_SIMPLE, double parameter = 0, int minimal_perimeter = 0, int recursive = 0 )
Approximates Freeman chain(s) with a polygonal curve.
This is a standalone contour approximation routine, not represented in the new interface. When cvFindContours retrieves contours as Freeman chains, it calls the function to get approximated contours, represented as polygons.
Parameters:
src_seq | Pointer to the approximated Freeman chain that can refer to other chains. |
storage | Storage location for the resulting polylines. |
method | Approximation method (see the description of the function :ocvFindContours ). |
parameter | Method parameter (not used now). |
minimal_perimeter | Approximates only those contours whose perimeters are not less than minimal_perimeter . Other chains are removed from the resulting structure. |
recursive | Recursion flag. If it is non-zero, the function approximates all chains that can be obtained from chain by using the h_next or v_next links. Otherwise, the single input chain is approximated. |
See also:
cvStartReadChainPoints, cvReadChainPoint
CvSeq* cvApproxPoly( const void* src_seq, int header_size, CvMemStorage* storage, int method, double eps, int recursive = 0 )
Approximates a single polygonal curve (contour) or a tree of polygonal curves (contours)
See also:
double cvArcLength( const void* curve, CvSlice slice = CV_WHOLE_SEQ, int is_closed = -1 )
Calculates perimeter of a contour or length of a part of contour.
See also:
CvRect cvBoundingRect( CvArr* points, int update = 0 )
Calculates contour bounding rectangle (update=1) or just retrieves pre-calculated rectangle (update=0)
See also:
void cvBoxPoints( CvBox2D box, CvPoint2D32f pt [4] )
Finds coordinates of the box vertices.
void cvCalcArrBackProject( CvArr** image, CvArr* dst, const CvHistogram* hist )
Calculates back project.
See also:
cvCalcBackProject, cv::calcBackProject
void cvCalcArrBackProjectPatch( CvArr** image, CvArr* dst, CvSize range, CvHistogram* hist, int method, double factor )
Locates a template within an image by using a histogram comparison.
The function calculates the back projection by comparing histograms of the source image patches with the given histogram. The function is similar to matchTemplate, but instead of comparing the raster patch with all its possible positions within the search window, the function CalcBackProjectPatch compares histograms. See the algorithm diagram below:
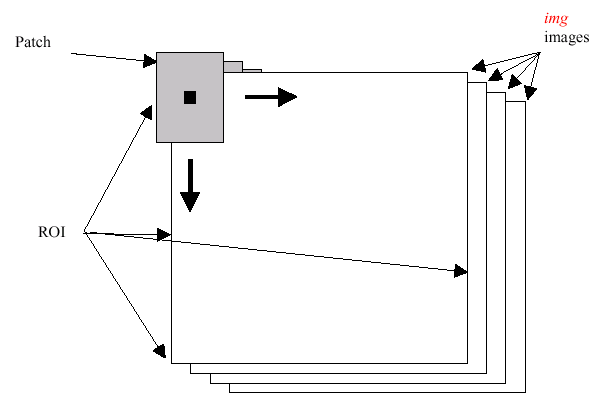
Parameters:
image | Source images (though, you may pass CvMat** as well). |
dst | Destination image. |
range | |
hist | Histogram. |
method | Comparison method passed to cvCompareHist (see the function description). |
factor | Normalization factor for histograms that affects the normalization scale of the destination image. Pass 1 if not sure. |
See also:
void cvCalcArrHist( CvArr** arr, CvHistogram* hist, int accumulate = 0, const CvArr* mask = NULL )
Calculates array histogram.
See also:
void cvCalcBayesianProb( CvHistogram** src, int number, CvHistogram** dst )
Calculates bayesian probabilistic histograms (each or src and dst is an array of number histograms.
float cvCalcEMD2( const CvArr* signature1, const CvArr* signature2, int distance_type, CvDistanceFunction distance_func = NULL, const CvArr* cost_matrix = NULL, CvArr* flow = NULL, float* lower_bound = NULL, void* userdata = NULL )
Computes earth mover distance between two weighted point sets (called signatures)
See also:
void cvCalcHist( IplImage** image, CvHistogram* hist, int accumulate = 0, const CvArr* mask = NULL )
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
void cvCalcProbDensity( const CvHistogram* hist1, const CvHistogram* hist2, CvHistogram* dst_hist, double scale = 255 )
Divides one histogram by another.
The function calculates the object probability density from two histograms as:
Parameters:
hist1 | First histogram (the divisor). |
hist2 | Second histogram. |
dst_hist | Destination histogram. |
scale | Scale factor for the destination histogram. |
void cvCanny( const CvArr* image, CvArr* edges, double threshold1, double threshold2, int aperture_size = 3 )
Runs canny edge detector.
See also:
int cvCheckContourConvexity(const CvArr* contour)
Checks whether the contour is convex or not (returns 1 if convex, 0 if not)
See also:
void cvCircle( CvArr* img, CvPoint center, int radius, CvScalar color, int thickness = 1, int line_type = 8, int shift = 0 )
Draws a circle with specified center and radius.
Thickness works in the same way as with cvRectangle
See also:
void cvClearHist(CvHistogram* hist)
Clears the histogram.
The function sets all of the histogram bins to 0 in case of a dense histogram and removes all histogram bins in case of a sparse array.
Parameters:
hist | Histogram. |
int cvClipLine( CvSize img_size, CvPoint* pt1, CvPoint* pt2 )
Clips the line segment connecting *pt1 and *pt2 by the rectangular window.
(0<=x<img_size.width, 0<=y<img_size.height).
See also:
CvScalar cvColorToScalar( double packed_color, int arrtype )
Unpacks color value.
if arrtype is CV_8UC?, color is treated as packed color value, otherwise the first channels (depending on arrtype) of destination scalar are set to the same value = color
double cvCompareHist( const CvHistogram* hist1, const CvHistogram* hist2, int method )
Compares two histogram
double cvContourArea( const CvArr* contour, CvSlice slice = CV_WHOLE_SEQ, int oriented = 0 )
Calculates area of a contour or contour segment.
See also:
double cvContourPerimeter(const void* contour)
same as cvArcLength for closed contour
void cvConvertMaps( const CvArr* mapx, const CvArr* mapy, CvArr* mapxy, CvArr* mapalpha )
Converts mapx & mapy from floating-point to integer formats for cvRemap.
See also:
CvSeq* cvConvexHull2( const CvArr* input, void* hull_storage = NULL, int orientation = CV_CLOCKWISE, int return_points = 0 )
Calculates exact convex hull of 2d point set.
See also:
CvSeq* cvConvexityDefects( const CvArr* contour, const CvArr* convexhull, CvMemStorage* storage = NULL )
Finds convexity defects for the contour.
See also:
void cvCopyHist( const CvHistogram* src, CvHistogram** dst )
Copies a histogram.
The function makes a copy of the histogram. If the second histogram pointer *dst is NULL, a new histogram of the same size as src is created. Otherwise, both histograms must have equal types and sizes. Then the function copies the bin values of the source histogram to the destination histogram and sets the same bin value ranges as in src.
Parameters:
src | Source histogram. |
dst | Pointer to the destination histogram. |
void cvCopyMakeBorder( const CvArr* src, CvArr* dst, CvPoint offset, int bordertype, CvScalar value = cvScalarAll(0) )
Copies source 2D array inside of the larger destination array and makes a border of the specified type (IPL_BORDER_*) around the copied area.
void cvCornerEigenValsAndVecs( const CvArr* image, CvArr* eigenvv, int block_size, int aperture_size = 3 )
Calculates eigen values and vectors of 2x2 gradient covariation matrix at every image pixel.
See also:
void cvCornerHarris( const CvArr* image, CvArr* harris_response, int block_size, int aperture_size = 3, double k = 0.04 )
Harris corner detector:
Calculates det(M) - k*(trace(M)^2), where M is 2x2 gradient covariation matrix for each pixel
See also:
void cvCornerMinEigenVal( const CvArr* image, CvArr* eigenval, int block_size, int aperture_size = 3 )
Calculates minimal eigenvalue for 2x2 gradient covariation matrix at every image pixel.
See also:
CvHistogram* cvCreateHist( int dims, int* sizes, int type, float** ranges = NULL, int uniform = 1 )
Creates a histogram.
The function creates a histogram of the specified size and returns a pointer to the created histogram. If the array ranges is 0, the histogram bin ranges must be specified later via the function cvSetHistBinRanges. Though cvCalcHist and cvCalcBackProject may process 8-bit images without setting bin ranges, they assume they are equally spaced in 0 to 255 bins.
Parameters:
dims | Number of histogram dimensions. |
sizes | Array of the histogram dimension sizes. |
type | Histogram representation format. CV_HIST_ARRAY means that the histogram data is represented as a multi-dimensional dense array CvMatND. CV_HIST_SPARSE means that histogram data is represented as a multi-dimensional sparse array CvSparseMat. |
ranges | Array of ranges for the histogram bins. Its meaning depends on the uniform parameter value. The ranges are used when the histogram is calculated or backprojected to determine which histogram bin corresponds to which value/tuple of values from the input image(s). |
uniform | Uniformity flag. If not zero, the histogram has evenly spaced bins and for every \(0<=i<cDims\) ranges[i] is an array of two numbers: lower and upper boundaries for the i-th histogram dimension. The whole range [lower,upper] is then split into dims[i] equal parts to determine the i-th input tuple value ranges for every histogram bin. And if uniform=0 , then the i-th element of the ranges array contains dims[i]+1 elements: \(\texttt{lower}_0, \texttt{upper}_0, \texttt{lower}_1, \texttt{upper}_1 = \texttt{lower}_2, ... \texttt{upper}_{dims[i]-1}\) where \(\texttt{lower}_j\) and \(\texttt{upper}_j\) are lower and upper boundaries of the i-th input tuple value for the j-th bin, respectively. In either case, the input values that are beyond the specified range for a histogram bin are not counted by cvCalcHist and filled with 0 by cvCalcBackProject. |
CvMat** cvCreatePyramid( const CvArr* img, int extra_layers, double rate, const CvSize* layer_sizes = 0, CvArr* bufarr = 0, int calc = 1, int filter = CV_GAUSSIAN_5x5 )
Builds pyramid for an image.
See also:
buildPyramid
IplConvKernel* cvCreateStructuringElementEx( int cols, int rows, int anchor_x, int anchor_y, int shape, int* values = NULL )
Returns a structuring element of the specified size and shape for morphological operations.
the created structuring element IplConvKernel* element must be released in the end using cvReleaseStructuringElement(&element)
.
Parameters:
cols | Width of the structuring element |
rows | Height of the structuring element |
anchor_x | x-coordinate of the anchor |
anchor_y | y-coordinate of the anchor |
shape | element shape that could be one of the cv::MorphShapes_c |
values | integer array of cols*rows elements that specifies the custom shape of the structuring element, when shape=CV_SHAPE_CUSTOM. |
See also:
void cvCvtColor( const CvArr* src, CvArr* dst, int code )
Converts input array pixels from one color space to another.
See also:
void cvDilate( const CvArr* src, CvArr* dst, IplConvKernel* element = NULL, int iterations = 1 )
dilates input image (applies maximum filter) one or more times.
If element pointer is NULL, 3x3 rectangular element is used
See also:
void cvDistTransform( const CvArr* src, CvArr* dst, int distance_type = CV_DIST_L2, int mask_size = 3, const float* mask = NULL, CvArr* labels = NULL, int labelType = CV_DIST_LABEL_CCOMP )
Applies distance transform to binary image.
See also:
void cvDrawContours( CvArr* img, CvSeq* contour, CvScalar external_color, CvScalar hole_color, int max_level, int thickness = 1, int line_type = 8, CvPoint offset = cvPoint(0, 0) )
Draws contour outlines or filled interiors on the image.
See also:
void cvEllipse( CvArr* img, CvPoint center, CvSize axes, double angle, double start_angle, double end_angle, CvScalar color, int thickness = 1, int line_type = 8, int shift = 0 )
Draws ellipse outline, filled ellipse, elliptic arc or filled elliptic sector.
depending on thickness, start_angle and end_angle parameters. The resultant figure is rotated by angle. All the angles are in degrees
See also:
int cvEllipse2Poly( CvPoint center, CvSize axes, int angle, int arc_start, int arc_end, CvPoint* pts, int delta )
Returns the polygon points which make up the given ellipse.
The ellipse is define by the box of size ‘axes’ rotated ‘angle’ around the ‘center’. A partial sweep of the ellipse arc can be done by spcifying arc_start and arc_end to be something other than 0 and 360, respectively. The input array ‘pts’ must be large enough to hold the result. The total number of points stored into ‘pts’ is returned by this function.
See also:
CvSeq* cvEndFindContours(CvContourScanner* scanner)
Releases contour scanner and returns pointer to the first outer contour.
See also:
void cvEqualizeHist( const CvArr* src, CvArr* dst )
equalizes histogram of 8-bit single-channel image
See also:
void cvErode( const CvArr* src, CvArr* dst, IplConvKernel* element = NULL, int iterations = 1 )
erodes input image (applies minimum filter) one or more times. If element pointer is NULL, 3x3 rectangular element is used
See also:
void cvFillConvexPoly( CvArr* img, const CvPoint* pts, int npts, CvScalar color, int line_type = 8, int shift = 0 )
Fills convex or monotonous polygon.
See also:
void cvFillPoly( CvArr* img, CvPoint** pts, const int* npts, int contours, CvScalar color, int line_type = 8, int shift = 0 )
Fills an area bounded by one or more arbitrary polygons.
See also:
void cvFilter2D( const CvArr* src, CvArr* dst, const CvMat* kernel, CvPoint anchor = cvPoint(-1,-1) )
Convolves an image with the kernel.
Parameters:
src | input image. |
dst | output image of the same size and the same number of channels as src. |
kernel | convolution kernel (or rather a correlation kernel), a single-channel floating point matrix; if you want to apply different kernels to different channels, split the image into separate color planes using split and process them individually. |
anchor | anchor of the kernel that indicates the relative position of a filtered point within the kernel; the anchor should lie within the kernel; default value (-1,-1) means that the anchor is at the kernel center. |
See also:
int cvFindContours( CvArr* image, CvMemStorage* storage, CvSeq** first_contour, int header_size = sizeof(CvContour), int mode = CV_RETR_LIST, int method = CV_CHAIN_APPROX_SIMPLE, CvPoint offset = cvPoint(0, 0) )
Retrieves outer and optionally inner boundaries of white (non-zero) connected components in the black (zero) background.
See also:
cv::findContours, cvStartFindContours, cvFindNextContour, cvSubstituteContour, cvEndFindContours
void cvFindCornerSubPix( const CvArr* image, CvPoint2D32f* corners, int count, CvSize win, CvSize zero_zone, CvTermCriteria criteria )
Adjust corner position using some sort of gradient search.
See also:
CvSeq* cvFindNextContour(CvContourScanner scanner)
Retrieves next contour.
See also:
CvBox2D cvFitEllipse2(const CvArr* points)
Fits ellipse into a set of 2d points.
See also:
void cvFitLine( const CvArr* points, int dist_type, double param, double reps, double aeps, float* line )
Fits a line into set of 2d or 3d points in a robust way (M-estimator technique)
See also:
void cvFloodFill( CvArr* image, CvPoint seed_point, CvScalar new_val, CvScalar lo_diff = cvScalarAll(0), CvScalar up_diff = cvScalarAll(0), CvConnectedComp* comp = NULL, int flags = 4, CvArr* mask = NULL )
Fills the connected component until the color difference gets large enough.
See also:
CvMat* cvGetAffineTransform( const CvPoint2D32f* src, const CvPoint2D32f* dst, CvMat* map_matrix )
Computes affine transform matrix for mapping src[i] to dst[i] (i=0,1,2)
See also:
double cvGetCentralMoment( CvMoments* moments, int x_order, int y_order )
Retrieve central moments.
void cvGetHuMoments( CvMoments* moments, CvHuMoments* hu_moments )
Calculates 7 Hu’s invariants from precalculated spatial and central moments.
See also:
void cvGetMinMaxHistValue( const CvHistogram* hist, float* min_value, float* max_value, int* min_idx = NULL, int* max_idx = NULL )
Finds the minimum and maximum histogram bins.
The function finds the minimum and maximum histogram bins and their positions. All of output arguments are optional. Among several extremas with the same value the ones with the minimum index (in the lexicographical order) are returned. In case of several maximums or minimums, the earliest in the lexicographical order (extrema locations) is returned.
Parameters:
hist | Histogram. |
min_value | Pointer to the minimum value of the histogram. |
max_value | Pointer to the maximum value of the histogram. |
min_idx | Pointer to the array of coordinates for the minimum. |
max_idx | Pointer to the array of coordinates for the maximum. |
double cvGetNormalizedCentralMoment( CvMoments* moments, int x_order, int y_order )
Retrieve normalized central moments.
CvMat* cvGetPerspectiveTransform( const CvPoint2D32f* src, const CvPoint2D32f* dst, CvMat* map_matrix )
Computes perspective transform matrix for mapping src[i] to dst[i] (i=0,1,2,3)
See also:
void cvGetQuadrangleSubPix( const CvArr* src, CvArr* dst, const CvMat* map_matrix )
Retrieves quadrangle from the input array.
matrixarr = ( a11 a12 | b1 ) dst(x,y) <- src(A[x y]’ + b) ( a21 a22 | b2 ) (bilinear interpolation is used to retrieve pixels with fractional coordinates)
See also:
void cvGetRectSubPix( const CvArr* src, CvArr* dst, CvPoint2D32f center )
Retrieves the rectangular image region with specified center from the input array.
dst(x,y) <- src(x + center.x - dst_width/2, y + center.y - dst_height/2). Values of pixels with fractional coordinates are retrieved using bilinear interpolation
See also:
double cvGetSpatialMoment( CvMoments* moments, int x_order, int y_order )
Retrieve spatial moments.
void cvGetTextSize( const char* text_string, const CvFont* font, CvSize* text_size, int* baseline )
Calculates bounding box of text stroke (useful for alignment)
See also:
void cvGoodFeaturesToTrack( const CvArr* image, CvArr* eig_image, CvArr* temp_image, CvPoint2D32f* corners, int* corner_count, double quality_level, double min_distance, const CvArr* mask = NULL, int block_size = 3, int use_harris = 0, double k = 0.04 )
Finds a sparse set of points within the selected region that seem to be easy to track.
See also:
CvSeq* cvHoughCircles( CvArr* image, void* circle_storage, int method, double dp, double min_dist, double param1 = 100, double param2 = 100, int min_radius = 0, int max_radius = 0 )
Finds circles in the image.
See also:
CvSeq* cvHoughLines2( CvArr* image, void* line_storage, int method, double rho, double theta, int threshold, double param1 = 0, double param2 = 0, double min_theta = 0, double max_theta = CV_PI )
Finds lines on binary image using one of several methods.
line_storage is either memory storage or 1 x max number of lines CvMat, its number of columns is changed by the function. method is one of CV_HOUGH_*; rho, theta and threshold are used for each of those methods; param1 ~ line length, param2 ~ line gap - for probabilistic, param1 ~ srn, param2 ~ stn - for multi-scale
See also:
void cvInitFont( CvFont* font, int font_face, double hscale, double vscale, double shear = 0, int thickness = 1, int line_type = 8 )
Initializes font structure (OpenCV 1.x API).
The function initializes the font structure that can be passed to text rendering functions.
Parameters:
font | Pointer to the font structure initialized by the function |
font_face | Font name identifier. See cv::HersheyFonts and corresponding old CV_* identifiers. |
hscale | Horizontal scale. If equal to 1.0f , the characters have the original width depending on the font type. If equal to 0.5f , the characters are of half the original width. |
vscale | Vertical scale. If equal to 1.0f , the characters have the original height depending on the font type. If equal to 0.5f , the characters are of half the original height. |
shear | Approximate tangent of the character slope relative to the vertical line. A zero value means a non-italic font, 1.0f means about a 45 degree slope, etc. |
thickness | Thickness of the text strokes |
line_type | Type of the strokes, see line description |
See also:
int cvInitLineIterator( const CvArr* image, CvPoint pt1, CvPoint pt2, CvLineIterator* line_iterator, int connectivity = 8, int left_to_right = 0 )
Initializes line iterator.
Initially, line_iterator->ptr will point to pt1 (or pt2, see left_to_right description) location in the image. Returns the number of pixels on the line between the ending points.
See also:
void cvInitUndistortMap( const CvMat* camera_matrix, const CvMat* distortion_coeffs, CvArr* mapx, CvArr* mapy )
Computes transformation map from intrinsic camera parameters that can used by cvRemap.
void cvInitUndistortRectifyMap( const CvMat* camera_matrix, const CvMat* dist_coeffs, const CvMat* R, const CvMat* new_camera_matrix, CvArr* mapx, CvArr* mapy )
Computes undistortion+rectification map for a head of stereo camera.
See also:
void cvIntegral( const CvArr* image, CvArr* sum, CvArr* sqsum = NULL, CvArr* tilted_sum = NULL )
Finds integral image: SUM(X,Y) = sum(x<X,y<Y)I(x,y)
See also:
void cvLaplace( const CvArr* src, CvArr* dst, int aperture_size = 3 )
Calculates the image Laplacian: (d2/dx + d2/dy)I.
See also:
void cvLine( CvArr* img, CvPoint pt1, CvPoint pt2, CvScalar color, int thickness = 1, int line_type = 8, int shift = 0 )
Draws 4-connected, 8-connected or antialiased line segment connecting two points.
See also:
void cvLinearPolar( const CvArr* src, CvArr* dst, CvPoint2D32f center, double maxRadius, int flags = CV_INTER_LINEAR+CV_WARP_FILL_OUTLIERS )
Performs forward or inverse linear-polar image transform
See also:
void cvLogPolar( const CvArr* src, CvArr* dst, CvPoint2D32f center, double M, int flags = CV_INTER_LINEAR+CV_WARP_FILL_OUTLIERS )
Performs forward or inverse log-polar image transform.
See also:
CvHistogram* cvMakeHistHeaderForArray( int dims, int* sizes, CvHistogram* hist, float* data, float** ranges = NULL, int uniform = 1 )
Makes a histogram out of an array.
The function initializes the histogram, whose header and bins are allocated by the user. cvReleaseHist does not need to be called afterwards. Only dense histograms can be initialized this way. The function returns hist.
Parameters:
dims | Number of the histogram dimensions. |
sizes | Array of the histogram dimension sizes. |
hist | Histogram header initialized by the function. |
data | Array used to store histogram bins. |
ranges | Histogram bin ranges. See cvCreateHist for details. |
uniform | Uniformity flag. See cvCreateHist for details. |
double cvMatchShapes( const void* object1, const void* object2, int method, double parameter = 0 )
Compares two contours by matching their moments.
See also:
void cvMatchTemplate( const CvArr* image, const CvArr* templ, CvArr* result, int method )
Measures similarity between template and overlapped windows in the source image and fills the resultant image with the measurements.
See also:
CvRect cvMaxRect( const CvRect* rect1, const CvRect* rect2 )
Finds minimum rectangle containing two given rectangles.
CvBox2D cvMinAreaRect2( const CvArr* points, CvMemStorage* storage = NULL )
Finds minimum area rotated rectangle bounding a set of points.
See also:
int cvMinEnclosingCircle( const CvArr* points, CvPoint2D32f* center, float* radius )
Finds minimum enclosing circle for a set of points.
See also:
void cvMoments( const CvArr* arr, CvMoments* moments, int binary = 0 )
Calculates all spatial and central moments up to the 3rd order.
See also:
void cvMorphologyEx( const CvArr* src, CvArr* dst, CvArr* temp, IplConvKernel* element, int operation, int iterations = 1 )
Performs complex morphological transformation.
See also:
void cvMultiplyAcc( const CvArr* image1, const CvArr* image2, CvArr* acc, const CvArr* mask = NULL )
Adds a product of two images to accumulator.
See also:
void cvNormalizeHist( CvHistogram* hist, double factor )
Normalizes the histogram.
The function normalizes the histogram bins by scaling them so that the sum of the bins becomes equal to factor.
Parameters:
hist | Pointer to the histogram. |
factor | Normalization factor. |
double cvPointPolygonTest( const CvArr* contour, CvPoint2D32f pt, int measure_dist )
Checks whether the point is inside polygon, outside, on an edge (at a vertex).
Returns positive, negative or zero value, correspondingly. Optionally, measures a signed distance between the point and the nearest polygon edge (measure_dist=1)
See also:
CvSeq* cvPointSeqFromMat( int seq_kind, const CvArr* mat, CvContour* contour_header, CvSeqBlock* block )
Initializes sequence header for a matrix (column or row vector) of points.
a wrapper for cvMakeSeqHeaderForArray (it does not initialize bounding rectangle!!!)
void cvPolyLine( CvArr* img, CvPoint** pts, const int* npts, int contours, int is_closed, CvScalar color, int thickness = 1, int line_type = 8, int shift = 0 )
Draws one or more polygonal curves.
See also:
void cvPreCornerDetect( const CvArr* image, CvArr* corners, int aperture_size = 3 )
Calculates constraint image for corner detection.
Dx^2 * Dyy + Dxx * Dy^2 - 2 * Dx * Dy * Dxy. Applying threshold to the result gives coordinates of corners
See also:
void cvPutText( CvArr* img, const char* text, CvPoint org, const CvFont* font, CvScalar color )
Renders text stroke with specified font and color at specified location. CvFont should be initialized with cvInitFont.
See also:
cvInitFont, cvGetTextSize, cvFont, cv::putText
void cvPyrDown( const CvArr* src, CvArr* dst, int filter = CV_GAUSSIAN_5x5 )
Smoothes the input image with gaussian kernel and then down-samples it.
dst_width = floor(src_width/2)[+1], dst_height = floor(src_height/2)[+1]
See also:
void cvPyrMeanShiftFiltering( const CvArr* src, CvArr* dst, double sp, double sr, int max_level = 1, CvTermCriteria termcrit = cvTermCriteria(CV_TERMCRIT_ITER+CV_TERMCRIT_EPS, 5, 1) )
Filters image using meanshift algorithm.
See also:
void cvPyrUp( const CvArr* src, CvArr* dst, int filter = CV_GAUSSIAN_5x5 )
Up-samples image and smoothes the result with gaussian kernel.
dst_width = src_width*2, dst_height = src_height*2
See also:
CvPoint cvReadChainPoint(CvChainPtReader* reader)
Retrieves the next chain point.
See also:
void cvRectangle( CvArr* img, CvPoint pt1, CvPoint pt2, CvScalar color, int thickness = 1, int line_type = 8, int shift = 0 )
Draws a rectangle given two opposite corners of the rectangle (pt1 & pt2)
if thickness<0 (e.g. thickness == CV_FILLED), the filled box is drawn
See also:
void cvRectangleR( CvArr* img, CvRect r, CvScalar color, int thickness = 1, int line_type = 8, int shift = 0 )
Draws a rectangle specified by a CvRect structure.
See also:
void cvReleaseHist(CvHistogram** hist)
Releases the histogram.
The function releases the histogram (header and the data). The pointer to the histogram is cleared by the function. If *hist pointer is already NULL, the function does nothing.
Parameters:
hist | Double pointer to the released histogram. |
void cvReleasePyramid( CvMat*** pyramid, int extra_layers )
Releases pyramid.
void cvReleaseStructuringElement(IplConvKernel** element)
releases structuring element
See also:
void cvRemap( const CvArr* src, CvArr* dst, const CvArr* mapx, const CvArr* mapy, int flags = CV_INTER_LINEAR+CV_WARP_FILL_OUTLIERS, CvScalar fillval = cvScalarAll(0) )
Performs generic geometric transformation using the specified coordinate maps.
See also:
void cvResize( const CvArr* src, CvArr* dst, int interpolation = CV_INTER_LINEAR )
Resizes image (input array is resized to fit the destination array)
See also:
void cvRunningAvg( const CvArr* image, CvArr* acc, double alpha, const CvArr* mask = NULL )
Adds image to accumulator with weights: acc = acc*(1-alpha) + image*alpha.
See also:
int cvSampleLine( const CvArr* image, CvPoint pt1, CvPoint pt2, void* buffer, int connectivity = 8 )
Fetches pixels that belong to the specified line segment and stores them to the buffer.
Returns the number of retrieved points.
See also:
void cvSetHistBinRanges( CvHistogram* hist, float** ranges, int uniform = 1 )
Sets the bounds of the histogram bins.
This is a standalone function for setting bin ranges in the histogram. For a more detailed description of the parameters ranges and uniform, see the :ocvCalcHist function that can initialize the ranges as well. Ranges for the histogram bins must be set before the histogram is calculated or the backproject of the histogram is calculated.
Parameters:
hist | Histogram. |
ranges | Array of bin ranges arrays. See :ocvCreateHist for details. |
uniform | Uniformity flag. See :ocvCreateHist for details. |
void cvSmooth( const CvArr* src, CvArr* dst, int smoothtype = CV_GAUSSIAN, int size1 = 3, int size2 = 0, double sigma1 = 0, double sigma2 = 0 )
Smooths the image in one of several ways.
Parameters:
src | The source image |
dst | The destination image |
smoothtype | Type of the smoothing, see SmoothMethod_c |
size1 | The first parameter of the smoothing operation, the aperture width. Must be a positive odd number (1, 3, 5, …) |
size2 | The second parameter of the smoothing operation, the aperture height. Ignored by CV_MEDIAN and CV_BILATERAL methods. In the case of simple scaled/non-scaled and Gaussian blur if size2 is zero, it is set to size1. Otherwise it must be a positive odd number. |
sigma1 | In the case of a Gaussian parameter this parameter may specify Gaussian \(\sigma\) (standard deviation). If it is zero, it is calculated from the kernel size:
\[\begin{split}\sigma = 0.3 (n/2 - 1) + 0.8 \quad \text{where} \quad n= \begin{array}{l l} \mbox{\texttt{size1} for horizontal kernel} \\ \mbox{\texttt{size2} for vertical kernel} \end{array}\end{split}\]
Using standard sigma for small kernels (\(3\times 3\) to \(7\times 7\)) gives better speed. If sigma1 is not zero, while size1 and size2 are zeros, the kernel size is calculated from the sigma (to provide accurate enough operation). |
sigma2 | additional parameter for bilateral filtering |
See also:
cv::GaussianBlur, cv::blur, cv::medianBlur, cv::bilateralFilter.
void cvSobel( const CvArr* src, CvArr* dst, int xorder, int yorder, int aperture_size = 3 )
Calculates an image derivative using generalized Sobel.
(aperture_size = 1,3,5,7) or Scharr (aperture_size = -1) operator. Scharr can be used only for the first dx or dy derivative
See also:
void cvSquareAcc( const CvArr* image, CvArr* sqsum, const CvArr* mask = NULL )
Adds squared image to accumulator.
See also:
CvContourScanner cvStartFindContours( CvArr* image, CvMemStorage* storage, int header_size = sizeof(CvContour), int mode = CV_RETR_LIST, int method = CV_CHAIN_APPROX_SIMPLE, CvPoint offset = cvPoint(0, 0) )
Initializes contour retrieving process.
Calls cvStartFindContours. Calls cvFindNextContour until null pointer is returned or some other condition becomes true. Calls cvEndFindContours at the end.
See also:
void cvStartReadChainPoints( CvChain* chain, CvChainPtReader* reader )
Initializes Freeman chain reader.
The reader is used to iteratively get coordinates of all the chain points. If the Freeman codes should be read as is, a simple sequence reader should be used
See also:
void cvSubstituteContour( CvContourScanner scanner, CvSeq* new_contour )
Substitutes the last retrieved contour with the new one.
(if the substitutor is null, the last retrieved contour is removed from the tree)
See also:
void cvThreshHist( CvHistogram* hist, double threshold )
Thresholds the histogram.
The function clears histogram bins that are below the specified threshold.
Parameters:
hist | Pointer to the histogram. |
threshold | Threshold level. |
double cvThreshold( const CvArr* src, CvArr* dst, double threshold, double max_value, int threshold_type )
Applies fixed-level threshold to grayscale image.
This is a basic operation applied before retrieving contours
See also:
void cvUndistort2( const CvArr* src, CvArr* dst, const CvMat* camera_matrix, const CvMat* distortion_coeffs, const CvMat* new_camera_matrix = 0 )
Transforms the input image to compensate lens distortion.
See also:
void cvUndistortPoints( const CvMat* src, CvMat* dst, const CvMat* camera_matrix, const CvMat* dist_coeffs, const CvMat* R = 0, const CvMat* P = 0 )
Computes the original (undistorted) feature coordinates from the observed (distorted) coordinates.
See also:
void cvWarpAffine( const CvArr* src, CvArr* dst, const CvMat* map_matrix, int flags = CV_INTER_LINEAR+CV_WARP_FILL_OUTLIERS, CvScalar fillval = cvScalarAll(0) )
Warps image with affine transform.
cvGetQuadrangleSubPix is similar to cvWarpAffine, but the outliers are extrapolated using replication border mode.
See also:
void cvWarpPerspective( const CvArr* src, CvArr* dst, const CvMat* map_matrix, int flags = CV_INTER_LINEAR+CV_WARP_FILL_OUTLIERS, CvScalar fillval = cvScalarAll(0) )
Warps image with perspective (projective) transform.
See also:
void cvWatershed( const CvArr* image, CvArr* markers )
Segments image using seed “markers”.
See also:
Macros
#define CV_INIT_3X3_DELTAS( \ deltas, \ step, \ nch \ )
initializes 8-element array for fast access to 3x3 neighborhood of a pixel