class cv::viz::WCameraPosition
Overview
This 3D Widget represents camera position in a scene by its axes or viewing frustum. : Moreā¦
#include <widgets.hpp> class WCameraPosition: public cv::viz::Widget3D { public: // construction WCameraPosition(double scale = 1.0); WCameraPosition( const Matx33d& K, double scale = 1.0, const Color& color = Color::white() ); WCameraPosition( const Vec2d& fov, double scale = 1.0, const Color& color = Color::white() ); WCameraPosition( const Matx33d& K, InputArray image, double scale = 1.0, const Color& color = Color::white() ); WCameraPosition( const Vec2d& fov, InputArray image, double scale = 1.0, const Color& color = Color::white() ); };
Inherited Members
public: // methods template <typename _W> _W cast(); Widget2D cast(); WWidgetMerger cast(); double getRenderingProperty(int property) const; Widget& operator=(const Widget& other); void setRenderingProperty( int property, double value ); static Widget fromPlyFile(const String& file_name); void applyTransform(const Affine3d& transform); Affine3d getPose() const; void setColor(const Color& color); void setPose(const Affine3d& pose); void updatePose(const Affine3d& pose);
Detailed Documentation
This 3D Widget represents camera position in a scene by its axes or viewing frustum. :
Construction
WCameraPosition(double scale = 1.0)
Creates camera coordinate frame at the origin.
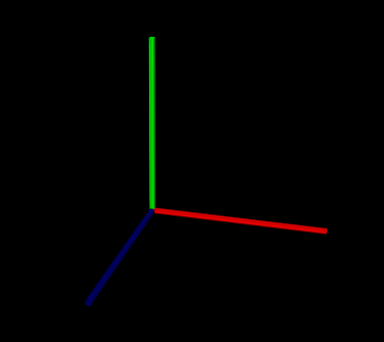
WCameraPosition( const Matx33d& K, double scale = 1.0, const Color& color = Color::white() )
Display the viewing frustum.
Creates viewing frustum of the camera based on its intrinsic matrix K.
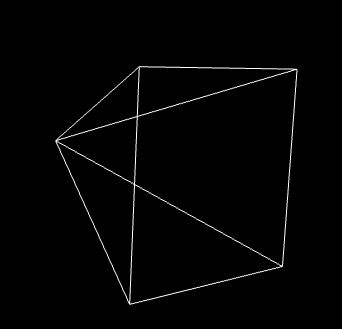
Parameters:
K | Intrinsic matrix of the camera. |
scale | Scale of the frustum. |
color | Color of the frustum. |
WCameraPosition( const Vec2d& fov, double scale = 1.0, const Color& color = Color::white() )
Display the viewing frustum.
Creates viewing frustum of the camera based on its field of view fov.
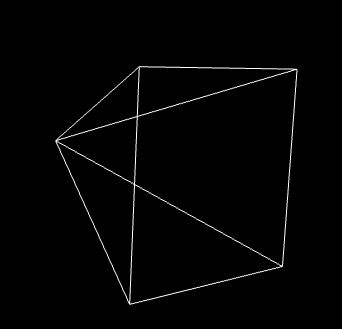
Parameters:
fov | Field of view of the camera (horizontal, vertical). |
scale | Scale of the frustum. |
color | Color of the frustum. |
WCameraPosition( const Matx33d& K, InputArray image, double scale = 1.0, const Color& color = Color::white() )
Display image on the far plane of the viewing frustum.
Creates viewing frustum of the camera based on its intrinsic matrix K, and displays image on the far end plane.
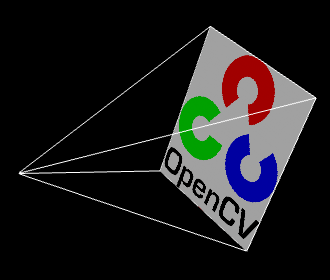
Parameters:
K | Intrinsic matrix of the camera. |
image | BGR or Gray-Scale image that is going to be displayed on the far plane of the frustum. |
scale | Scale of the frustum and image. |
color | Color of the frustum. |
WCameraPosition( const Vec2d& fov, InputArray image, double scale = 1.0, const Color& color = Color::white() )
Display image on the far plane of the viewing frustum.
Creates viewing frustum of the camera based on its intrinsic matrix K, and displays image on the far end plane.
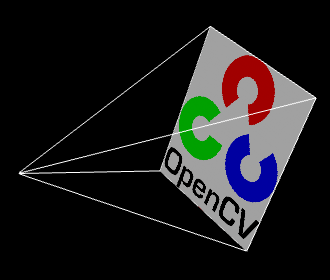
Parameters:
fov | Field of view of the camera (horizontal, vertical). |
image | BGR or Gray-Scale image that is going to be displayed on the far plane of the frustum. |
scale | Scale of the frustum and image. |
color | Color of the frustum. |